Logging
Logging is important in order to be able to follow up on the execution of a particular Job. The log To use the logger API, see examples below.
context.logger().info('Hello from script!')
context.logger().warning('Bad value %s received...', badValue)
context.logger().error('This is an error');
context.logger().debug('This message is only shown if DEBUG explicitly have been enabled via the Admin UI')
Console API
When developing scripts for the NodeJS platform, or Web browsers, the Console API is commonly used to perform logging from an application.
A subset of the Console API is also available when using the JS Executor in TIF.
A global object called console
is bound to the script.
Mainly these functions acts as wrapper calls for the Logger APIs availabe in the Execution Context.
The example below shows which of these methods that are available from the console API in TIF.
// Various logging methods: (1)
console.info('Hello from javascript');
console.log('Same as using info');
console.warn('A warning message');
console.error('An error message');
console.debug('Only visible if DEBUG is enabled');
console.trace('Same as using debug');
// Time related (2)
console.time(); // starts the default timer
console.time('Custom'); // starts the Custom timer
console.timeLog(); // logs the time since start for the default timer
console.timeLog('Custom'); // logs the Custom timer
console.timeEnd(); // Ends the default timer
console.timeEnd('Custom'); // Ends the Custom timer
1 | The methods supports using %s , %o , %i , %d , %f formatting options as described in the Console API from the Mozilla documentation. NOTE: These formatting options follows the Javascript specification and is not behaving the same as when using the logger API directly from the ExecutionContext (context.logger()). |
2 | The timer related methods will log standard messages using the INFO level per default. Any faulty usage, such as trying to log a non-existing timer, will be logged using the WARN level. |
Thresholds
To not cause performance issues of the application, there are some restrictions on how much data you can log from one Job execution.
If the amount of logging data exceeds one or both of the below criteria, the logging in the UI is suspended and the complete log is attached as a Job Data File to the Job instead.
-
Maximum nr of log entries: 150
-
Total nr of characters logged: 100k
Also note that if a single log message exceeds 1k characters, it will be truncated.
Debug Logging
To both make the logs from a job understandable and at the same time not fill the logs with unnecessary information (also revealing secrets / tokens etc), there is a "debug" method on the Logger available during the execution.
The information written using the "debug" log level will ONLY be persisted IF the DEBUG flag in your TIF Environment is enabled. If it is disabled, debug messages are not captured.
These are the API methods to use:
context.logger().debug('<log statement goes here>', optionalArgs);
// OR using the console API (console.debug or console.trace)
console.debug('<log statemenet>', optionalArgs)
And you can also test if logging is enabled via:
if (context.logger().isDebugEnabled()) {
// code
}
To enable DEBUG, you go to this page and enable it:
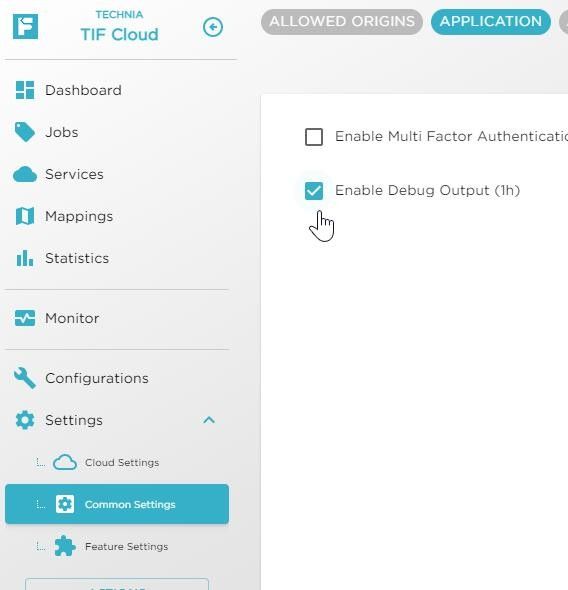
In order to not swamp the system with debug messages, the debug output is enabled for 1 hour after saving the changes. The debug level is turned off automatically when this time expires.